Hello everyone! In this guide, we’ll explore the practical aspects of managing VHDX files using PowerShell. Our journey will cover a range of topics, from setting up your PowerShell environment and executing basic file management commands to automating routine tasks with scripts. We’ll also delve into performance optimization techniques for VHDX files and address common troubleshooting scenarios. By providing step-by-step instructions and actionable scripts, this article aims to enhance your efficiency and expertise in handling VHDX files through PowerShell.
Setting Up Your PowerShell Environment
Before diving into VHDX file management, ensuring your PowerShell environment is correctly set up is crucial. This setup involves having the right PowerShell version and necessary modules installed. PowerShell 5.1 or later is recommended for the best compatibility with the scripts and commands we’ll discuss.
How to Check Your PowerShell Version:
- Open PowerShell as an administrator.
- Type
$PSVersionTable.PSVersion
and press Enter. This command displays your current PowerShell version. - If your version is below 5.1, consider upgrading to a newer version for improved functionality and security.
Installing Required Modules: For managing VHDX files, you’ll need the Hyper-V PowerShell module. This module is typically included with Windows 10 and Windows Server editions but may require manual installation or enabling on some systems.
To Install or Enable the Hyper-V Module:
- Open the Control Panel.
- Navigate to “Programs and Features” > “Turn Windows features on or off.”
- Scroll down to “Hyper-V” and check the box next to “Hyper-V Management Tools.”
- Click OK and wait for the installation to complete. You might need to restart your computer.
Once your environment is set up, you’re ready to start managing VHDX files with PowerShell commands and scripts.
Basic PowerShell Commands for VHDX Management
With your environment set up, let’s cover some basic commands to start managing VHDX files:
1. Listing VHDX Files:
- To list all VHDX files in a specific directory, use:
Get-ChildItem -Path C:\PathToYourVHDXFiles\ -Filter *.vhdx
.- Replace
C:\PathToYourVHDXFiles\
with the actual path where your VHDX files are stored.
- Replace
2. Creating a New VHDX File:
- Use the
New-VHD
command to create a new VHDX file:New-VHD -Path C:\PathToYourVHDXFiles\YourNewVHDX.vhdx -SizeBytes 50GB
.- This command creates a new VHDX file named
YourNewVHDX.vhdx
with a size of 50GB at the specified path.
- This command creates a new VHDX file named
3. Mounting a VHDX File:
- To mount a VHDX file to a local drive, use:
Mount-VHD -Path C:\PathToYourVHDXFiles\YourVHDXFile.vhdx
.- This makes the VHDX file accessible as a local drive, allowing you to interact with it as you would with any physical disk.
4. Compacting a VHDX File:
- VHDX files can grow larger than the data they contain. To compact a VHDX file, first, ensure it’s not mounted, then use:
Optimize-VHD -Path C:\PathToYourVHDXFiles\YourVHDXFile.vhdx -Mode Full
.- This reduces the file size by removing unused space within the VHDX file.
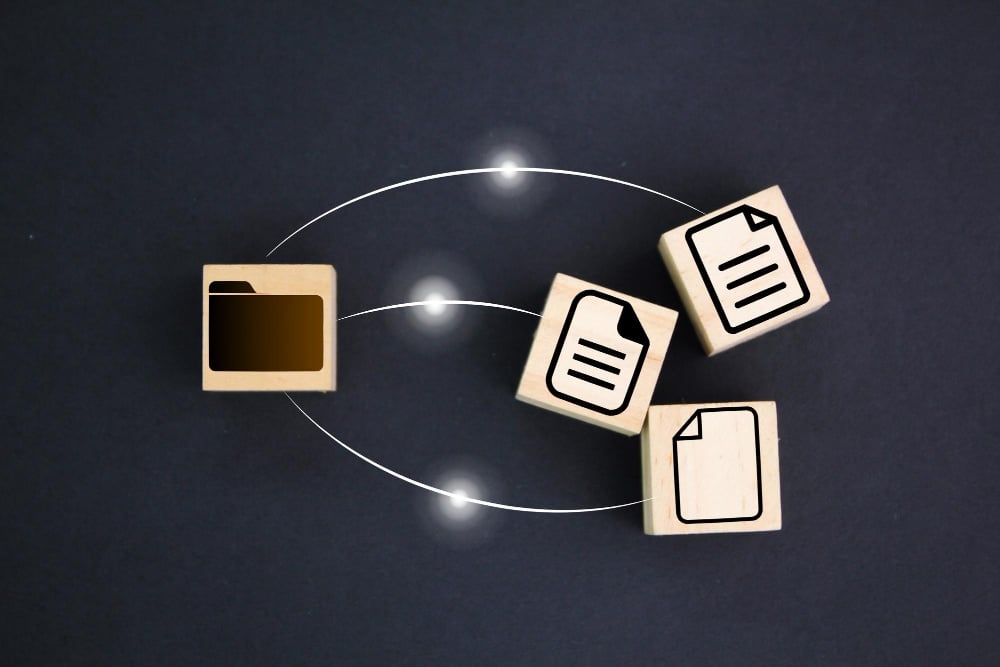
Automating VHDX Tasks with PowerShell Scripts
Automation through PowerShell can significantly enhance efficiency when managing VHDX files. Beyond simple tasks, scripting allows for the execution of complex management routines with ease. Let’s delve into a more detailed script example that showcases automation of several tasks, including checking for disk space, creating, and maintaining VHDX files.
Script Example: Automated VHDX Management
This script automates the creation, mounting, checking of disk space, and cleanup of VHDX files based on predefined criteria:
# Define the path for VHDX storage
$vhdxStoragePath = "C:\VHDXStorage"
# Create a new VHDX file if it doesn't exist
$vhdxFilePath = "$vhdxStoragePath\MyVHDX.vhdx"
if (-not (Test-Path $vhdxFilePath)) {
New-VHD -Path $vhdxFilePath -SizeBytes 100GB | Mount-VHD -Passthru | Initialize-Disk -Passthru | New-Partition -AssignDriveLetter -UseMaximumSize | Format-Volume -FileSystem NTFS -Confirm:$false
Write-Output "VHDX file created and formatted."
}
# Function to check and maintain disk space
Function CheckAndMaintainDiskSpace {
Param (
[string]$vhdxFile
)
$diskInfo = Get-VHD -Path $vhdxFile | Get-Disk
$freeSpacePercentage = ($diskInfo.Size - $diskInfo.AllocatedSize) / $diskInfo.Size * 100
if ($freeSpacePercentage -lt 20) {
Optimize-VHD -Path $vhdxFile -Mode Full
Write-Output "VHDX file optimized to free up space."
}
}
# Automate maintenance tasks
CheckAndMaintainDiskSpace -vhdxFile $vhdxFilePath
# Schedule this script to run regularly for continuous management
# Note: Scheduling can be done through Task Scheduler or similar tools, using PowerShell to trigger this script.
Explanation of the Script
- VHDX Creation and Initialization: The script starts by checking if a VHDX file exists at the specified location. If not, it creates a 100GB VHDX file, mounts it, initializes the disk, creates a partition, assigns a drive letter, and formats it with the NTFS file system.
- Disk Space Maintenance: The
CheckAndMaintainDiskSpace
function checks the VHDX file’s free space percentage. If free space drops below 20%, the script optimizes the VHDX file to reclaim unused space, ensuring efficient storage use. - Scheduling for Regular Maintenance: The script includes a placeholder for scheduling regular maintenance tasks. This ensures that VHDX files are consistently managed without manual intervention, using Task Scheduler or similar tools to automate the script’s execution.
Optimizing VHDX Performance with PowerShell
Performance optimization of VHDX files is critical for maintaining fast and reliable access to virtual disks. Here are some PowerShell techniques to enhance VHDX performance:
1. Dynamic vs. Fixed VHDX Files:
- Fixed VHDX files allocate all specified disk space at creation, which can enhance performance since the virtual disk doesn’t need to expand during operation. However, it uses more physical storage upfront.
- Dynamic VHDX files allocate space as needed, up to the maximum size. While this approach is storage-efficient, it can lead to fragmentation and slower performance over time.
Converting Dynamic to Fixed VHDX for Performance:
Convert-VHD -Path "C:\VHDXStorage\DynamicVHDX.vhdx" -DestinationPath "C:\VHDXStorage\FixedVHDX.vhdx" -VHDType Fixed
This command converts a dynamic VHDX file to a fixed one, potentially enhancing performance by avoiding runtime expansion.
2. VHDX Defragmentation: Fragmentation can significantly impact the performance of VHDX files. While Windows automatically defragments physical drives, VHDX files might require manual defragmentation.
Defragmenting a VHDX File:
Optimize-Volume -DriveLetter X -ReTrim -Defrag -SlabConsolidate -TierOptimize
Replace X
with the drive letter of the mounted VHDX. This command optimizes the volume by defragmenting files, consolidating slabs (in tiered storage), and optimizing tiers.
We’ve covered a lot about managing VHDX files with PowerShell in this guide. From getting your setup right, through basic commands, to some pretty neat automation tricks, and even how to keep things running smoothly. It’s a lot, but it’s all aimed at making your work with VHDX files easier and more efficient.
Now it’s your turn! We’d love to hear from you. Got a question, a cool tip, or maybe you ran into a hiccup trying something out? Drop us a comment or send your thoughts our way. If there’s something specific you want to know more about, or if you have suggestions for future topics, let us know. Your feedback not only helps us get better but also helps us tailor our content to what you really need.