The Need for Automated VBM Management
VBM files play a crucial role in tracking backup consistency and restoring processes. Manual management of VBM files not only consumes valuable time but also introduces a margin for error that can jeopardize data integrity. Automation, through PowerShell and Veeam APIs, emerges as a powerful solution to these challenges. It offers a way to streamline VBM file management, enhancing both reliability and efficiency. This guide delves into the steps necessary to automate VBM file management, paving the way for a more resilient backup strategy.
Prerequisites for Automation with PowerShell and Veeam APIs
Before diving into automation, it’s essential to establish a solid foundation. Ensure that you have the following prerequisites in place:
- Veeam Backup & Replication: Verify that you’re running a version that supports API interactions. Typically, newer versions offer more extensive API capabilities.
- PowerShell: Basic knowledge of PowerShell scripting is required. Familiarize yourself with PowerShell cmdlets and scripting fundamentals.
- Access Permissions: Administrative privileges on the Veeam Backup & Replication server are necessary. Additionally, ensure API access is enabled and properly configured.
- Development Environment: While not mandatory, having a dedicated development environment for testing your scripts can prevent unintended disruptions in your production environment.
Once these prerequisites are met, you’re ready to embark on the journey of automating VBM file management. The next sections will guide you through connecting PowerShell to Veeam APIs, executing automation tasks, and refining your scripts for optimal performance.
Connecting PowerShell to Veeam APIs
To harness the power of automation in managing VBM files, establishing a connection between PowerShell and Veeam’s API is essential. This connection enables scripts to perform operations such as querying backup job details, managing backup files, and automating routine tasks directly through the Veeam Backup & Replication server.
API Endpoint Configuration:
- First, identify the Veeam Backup & Replication server’s API endpoint. This is typically the server’s URL followed by “/api/”. For example, if your Veeam server is accessible at
https://veeamserver.example.com
, the API endpoint would behttps://veeamserver.example.com/api/
.
Authenticating with the Veeam Server:
- To execute API calls, you must authenticate with the Veeam server. PowerShell makes this easy with the
Invoke-RestMethod
cmdlet. Use it to send a POST request to the/sessionMngr/?v=latest
endpoint, including your Veeam server credentials in the request header. - Here’s an example script snippet for authentication:
$credentials = Get-Credential
$base64AuthInfo = [Convert]::ToBase64String([Text.Encoding]::ASCII.GetBytes(("{0}:{1}" -f $credentials.UserName, $credentials.GetNetworkCredential().Password)))
$headers = @{
"Authorization" = "Basic $base64AuthInfo"
"X-RestSvcSessionId" = ""
}
$session = Invoke-RestMethod -Uri "https://veeamserver.example.com/api/sessionMngr/?v=latest" -Method Post -Headers $headers
$headers["X-RestSvcSessionId"] = $session.SessionId
Making API Calls:
- With authentication set, you can now make API calls to manage VBM files. For instance, to list all backup jobs, you might use the
/query?type=BackupJob
endpoint. - An example API call to list backup jobs:
$backupJobs = Invoke-RestMethod -Uri "https://veeamserver.example.com/api/query?type=BackupJob" -Method Get -Headers $headers
foreach ($job in $backupJobs) {
Write-Output "Job Name: $($job.Name)"
}
By establishing a connection between PowerShell and Veeam’s API, you’ve laid the groundwork for automating VBM file management. This setup allows for the execution of complex management tasks with the simplicity and efficiency of PowerShell scripts.
Automating VBM File Management Tasks
With a connection between PowerShell and Veeam APIs established, automating the management of VBM files becomes straightforward. These files, crucial for the integrity of backup operations, can be efficiently managed through scripting. Here, we focus on automation scripts for key tasks such as creation, maintenance, and deletion of VBM files.
Creating Backup Jobs with Custom VBM Management
Automating the creation of backup jobs involves not just initiating the job but also managing the resulting VBM files. This script sample demonstrates how to create a backup job and implement a naming convention for VBM files:
# Example script to create a backup job and manage VBM file naming
$backupJobParams = @{
"Name" = "Daily Backup Job"
"Description" = "Automated backup job with custom VBM management"
# Additional parameters for the backup job
}
$newBackupJob = Invoke-RestMethod -Uri "https://veeamserver.example.com/api/backupJobs" -Method Post -Headers $headers -Body $backupJobParams
# Implement custom VBM file management logic here
# For example, renaming the VBM file according to a specific convention
Automating VBM File Cleanup
Regular maintenance of VBM files ensures your backup environment remains organized and free from clutter. This script demonstrates how to identify and delete VBM files associated with deleted or obsolete backup jobs:
# Example script to clean up VBM files for deleted backup jobs
$allBackupJobs = Invoke-RestMethod -Uri "https://veeamserver.example.com/api/backupJobs" -Method Get -Headers $headers
foreach ($job in $allBackupJobs) {
if ($job.Status -eq "Deleted") {
$vbmFilePath = "<Path to VBM Files>/" + $job.Name + ".vbm"
Remove-Item -Path $vbmFilePath -Force
}
}
Managing VBM File Integrity
Ensuring the integrity of VBM files is critical. This script outlines a method for verifying the integrity of VBM files, potentially alerting administrators to any issues:
# Example script to verify the integrity of VBM files
$allVBMFiles = Get-ChildItem -Path "<Path to VBM Files>" -Filter *.vbm
foreach ($vbmFile in $allVBMFiles) {
# Implement integrity check logic here
# This could involve verifying file size, last modified date, or a checksum
}
These examples demonstrate the power of automating VBM file management with PowerShell and Veeam APIs. By leveraging these scripts, administrators can significantly reduce manual effort, enhance efficiency, and maintain high standards of data integrity.
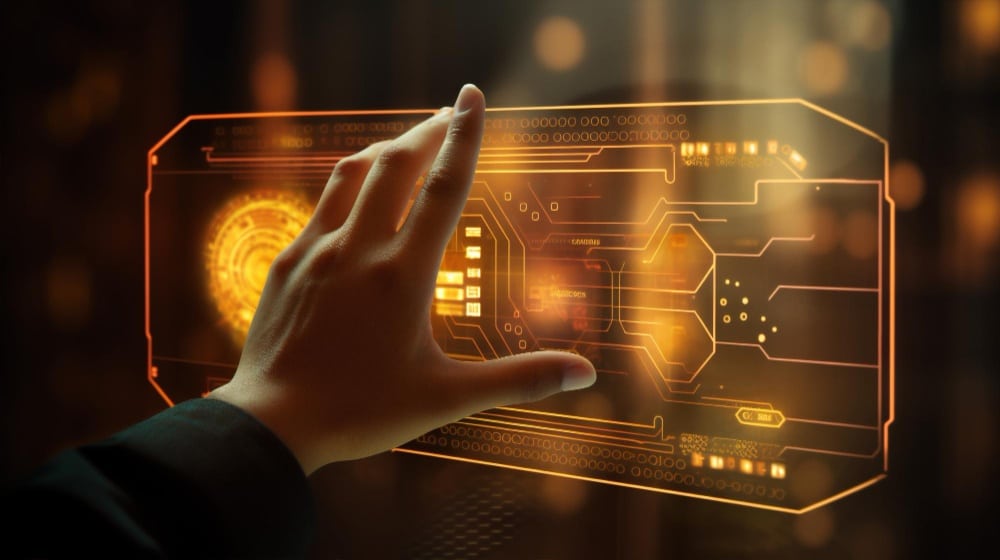
Error Handling and Logging in Automation
Automating VBM file management with PowerShell and Veeam APIs introduces a level of complexity that necessitates robust error handling and detailed logging. These practices ensure that any problems encountered during execution are caught and addressed, and that there’s a comprehensive record of actions taken by the scripts.
- Implementing Try-Catch Blocks:
- PowerShell’s try-catch blocks are essential for catching exceptions that occur during script execution. By wrapping your API calls in these blocks, you can specifically handle different types of errors, whether they are network issues, authentication failures, or API rate limits.
- Example of using a try-catch block:
try {
# Attempt to make an API call
$response = Invoke-RestMethod -Uri $apiUrl -Method Post -Headers $headers
# Process the response
} catch {
# Log error details
Write-Output "An error occurred: $_"
}
Logging Operations and Errors:
- Effective logging is crucial for troubleshooting and auditing your automated tasks. PowerShell can easily log to a file, event viewer, or even a central logging platform. Choose a method that fits your environment and ensures that logs are accessible and meaningful.
- Script snippet for logging to a file:
$logPath = "C:\Logs\VeeamAutomationLog.txt"
# Example log entry
$logEntry = "Backup job created successfully at $(Get-Date)"
Add-Content -Path $logPath -Value $logEntry
Error Recovery Strategies:
- In some cases, it’s possible to automate recovery actions in response to certain errors. For example, if a backup job creation fails due to a timeout, the script could retry the operation automatically.
- Implementing a retry mechanism:
$retryCount = 0
$success = $false
while (-not $success -and $retryCount -lt 3) {
try {
# Attempt to perform the operation
$retryCount++
# If successful, set $success to $true
} catch {
Write-Output "Attempt $retryCount failed: $_"
Start-Sleep -Seconds 5
}
}
Incorporating thorough error handling and logging into your automation scripts is non-negotiable. It not only aids in identifying and resolving issues more efficiently but also contributes to the scripts’ overall resilience and reliability.
Optimizing Your VBM Automation Scripts
Efficient automation scripts are the backbone of a streamlined VBM file management process. Optimization involves not just enhancing the performance of individual scripts but also ensuring they operate harmoniously within your IT ecosystem. Here are strategies to achieve this:
Minimizing API Calls:
- Each API call consumes resources and time. Where possible, consolidate API calls or fetch data in bulk to reduce the load on your network and the Veeam server. This can also help avoid hitting rate limits on the API.
- Example optimization:
# Instead of calling the API for each job, fetch all jobs and filter locally
$allJobs = Invoke-RestMethod -Uri $jobsUrl -Method Get -Headers $headers
$relevantJobs = $allJobs | Where-Object { $_.SomeCriteria -eq "Value" }
Script Scheduling and Throttling:
- Run scripts during off-peak hours to minimize the impact on network and server performance. Additionally, consider implementing throttling in your scripts to space out operations, especially if you’re working with a large number of VBM files.
- Scheduling example using Task Scheduler or cron jobs, depending on your operating system.
Error Handling Efficiency:
- While error handling is crucial, it’s also important to ensure that your error-handling logic doesn’t introduce unnecessary delays. Use specific catch blocks for known errors and a general catch block for unexpected errors to streamline processing.
- Example of efficient error handling:
try {
# Attempt operation
} catch [SpecificException] {
# Handle known exception efficiently
} catch {
# Fallback error handling
}
Regular Script Review and Refactoring:
- As your environment and Veeam’s API evolve, regularly review and refactor your scripts. Removing obsolete functions, updating API endpoints, and revising logic can lead to significant performance improvements.
- Consider code reviews with peers to identify optimization opportunities.
Utilizing PowerShell Parallel Processing:
- For tasks that can be executed in parallel, such as processing multiple VBM files simultaneously, leverage PowerShell’s parallel processing capabilities to reduce overall execution time.
- Example using
ForEach-Object -Parallel
(requires PowerShell 7 or higher):
$jobList | ForEach-Object -Parallel {
# Process each job in parallel
}
By applying these optimization techniques, you can ensure that your automation scripts are not only effective but also resource-efficient and scalable. This will lead to smoother operations and a more resilient backup management process.
Security Considerations in Automation
Automating the management of VBM files with PowerShell and Veeam APIs introduces several security considerations that must be addressed to safeguard your backup infrastructure. Here are essential security practices to implement:
Secure Credential Management:
- Avoid hard-coding credentials in scripts. Use secure storage solutions like PowerShell’s
Get-Credential
cmdlet, Windows Credential Manager, or external secrets managers to retrieve credentials at runtime. - Example using
Get-Credential
:
$credentials = Get-Credential
Implement Role-Based Access Control (RBAC):
- Limit API access to only those users or services that require it to perform their tasks. This principle of least privilege reduces the risk of unauthorized actions.
- Configure RBAC settings within Veeam and your operating system to control access based on roles.
Use HTTPS for API Communications:
- Ensure that all communications with the Veeam API over the network are encrypted using HTTPS. This prevents eavesdropping and the interception of sensitive data during transmission.
- Verify that your Veeam server’s API endpoint is configured to use HTTPS.
Regularly Update and Patch:
- Keep your Veeam Backup & Replication, PowerShell, and operating system up to date with the latest security patches. This protects against vulnerabilities that could be exploited by attackers.
- Schedule regular maintenance windows for updates.
Audit and Monitor Script Executions:
- Implement logging and monitoring for all script executions to detect unusual activities or unauthorized access attempts. Use tools like Windows Event Viewer or syslog servers for centralized logging.
- Example logging script actions:
Write-EventLog -LogName Application -Source "VBM Automation" -EntryType Information -EventId 1 -Message "Script executed successfully."
Secure Script Files and Sensitive Data:
- Store scripts and any files containing sensitive information in secure locations with restricted access. Use file system permissions to control who can read or execute the scripts.
- Encrypt sensitive data at rest to protect it from unauthorized access.
By adhering to these security best practices, you can ensure that your automation efforts enhance your backup management processes without introducing additional risks. It’s crucial to maintain a security-first approach throughout the automation lifecycle, from development to deployment and maintenance.